Accessing DALL-E Through OpenAI’s API
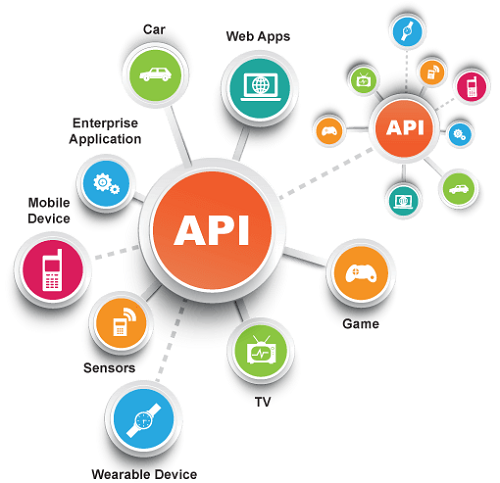
- API Key: Ensure you have an API key from OpenAI. This typically involves signing up for an API account on the OpenAI website.
- API Documentation: Familiarize yourself with the API documentation provided by OpenAI. This typically contains details on the various endpoints, how to authenticate, and the kind of data you can send or receive. As of writing, they periodically update the availability and access scopes for different models.
- Endpoint URLs: To access DALL-E, you might need a specific endpoint URL designed for image generation tasks. You can find this in the API documentation or by contacting OpenAI support.
- Setup Your Environment: You can use programming languages like Python to interact with the API. Here’s a simple setup in Python:
- import openai
- # Load your API key from an environment variable or secret management service
- openai.api_key = “your-api-key-here”
- def generate_image(prompt):
- response = openai.Image.create(
- prompt=prompt,
- n=1,
- size=”1024×1024″
- )
- image_url = response[‘data’][0][‘url’]
- return image_url
- prompt = “A futuristic city skyline at sunset”
- image_url = generate_image(prompt)
- print(f”Generated image URL: {image_url}”)
- Handle Responses: The API will return data in JSON format, including the URL of the generated image. Ensure you can handle and display it appropriately in your application.
Important Points to Consider
- Rate Limits: Be aware of the rate limits imposed on the API calls. Exceeding these limits might result in temporary bans or throttling.
- Costs: Generating images can be more compute-intensive than text and might incur higher usage costs. Monitor your usage to avoid unexpected charges.
- Permissions: You might need specific permissions to access certain features of the API. Always check the latest updates in the API documentation or your account’s usage details.
Additional Resources - API Documentation: OpenAI API Documentation
- Community Support: Engage with the OpenAI community for troubleshooting and tips.
- Updates: Keep an eye on OpenAI’s announcements for new features or changes to access policies.
If you encounter any issues or have specific questions regarding the API’s capabilities, it’s often helpful to contact OpenAI support directly for the most accurate and personalized assistance.